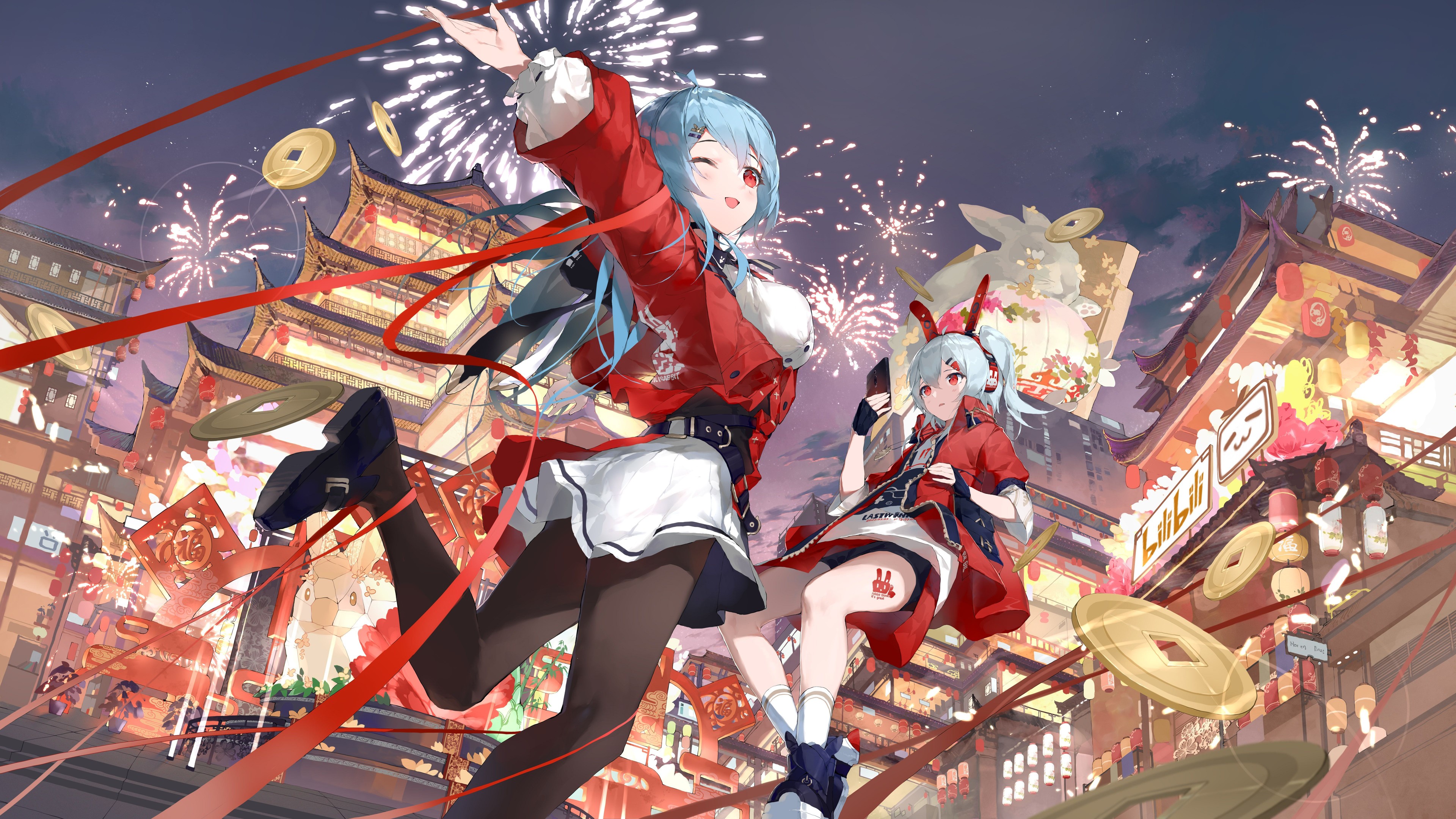
2023-2024 (2) 数据结构题目集
AkariPTA - 2023-2024(2)数据结构题目集
6-01 顺序表操作集
本题要求实现顺序表的操作集。
题目描述
函数接口定义:
List MakeEmpty(); |
其中List
结构定义如下:
typedef int Position; |
各个操作函数的定义为:
List MakeEmpty()
:创建并返回一个空的线性表;
Position Find( List L, ElementType X )
:返回线性表中X的位置。若找不到则返回ERROR;
bool Insert( List L, ElementType X, Position P )
:将X插入在位置P并返回true。若空间已满,则打印“FULL”并返回false;如果参数P指向非法位置,则打印“ILLEGAL POSITION”并返回false;
bool Delete( List L, Position P )
:将位置P的元素删除并返回true。若参数P指向非法位置,则打印“POSITION P EMPTY”(其中P是参数值)并返回false。
输入样例:
6
1 2 3 4 5 6
3
6 5 1
2
-1 6
输出样例:
FULL Insertion Error: 6 is not in.
Finding Error: 6 is not in.
5 is at position 0.
1 is at position 4.
POSITION -1 EMPTY Deletion Error.
FULL Insertion Error: 0 is not in.
POSITION 6 EMPTY Deletion Error.
FULL Insertion Error: 0 is not in.
List MakeEmpty(){ |
6-02 线性表元素的区间删除
给定一个顺序存储的线性表,请设计一个函数删除所有值大于 min 而且小于 max 的元素。删除后表中剩余元素保持顺序存储,并且相对位置不能改变。
题目描述
函数接口定义:
List Delete( List L, ElementType minD, ElementType maxD ); |
其中List结构定义如下:
typedef int Position; |
L是用户传入的一个线性表,其中ElementType元素可以通过>、==、<进行比较;minD和maxD分别为待删除元素的值域的下、上界。函数Delete应将Data[]中所有值大于minD而且小于maxD的元素删除,同时保证表中剩余元素保持顺序存储,并且相对位置不变,最后返回删除后的表。
输入样例:
10
4 -8 2 12 1 5 9 3 3 10
0 4
输出样例:
4 -8 12 5 9 10
大致思路:
先标记满足要求的元素,再重新整理数组,将不满足要求的元素保留在原数组中,最后更新 Last 的值来反映删除元素后的数组长度。
// 要求: 满足的删除, 不满足的保留 |
6-03 递增的整数序列链表的插入
本题要求实现一个函数,在递增的整数序列链表(带头结点)中插入一个新整数,并保持该序列的有序性。
题目描述
函数接口定义:
List Insert( List L, ElementType X ); |
其中List结构定义如下:
typedef struct Node *PtrToNode; |
L是给定的带头结点的单链表,其结点存储的数据是递增有序的;函数Insert要将X插入L,并保持该序列的有序性,返回插入后的链表头指针。
输入样例:
5
1 2 4 5 6
3
输出样例:
1 2 3 4 5 6
大致思路:
- 因为是严格递增的序列, 所以省了很多事, 不需要找
pos
位置什么的了. - 依然要判断 p 是否为空, 即
p->Next != NULL
, 可简化为while (p->Next)
List Insert( List L, ElementType X ){ |